If you try to make a API call in xamarin, you should use the HTTPClient
, this is a standard network call for c#, but when you try to call with SSL on Android, you will get the below error:
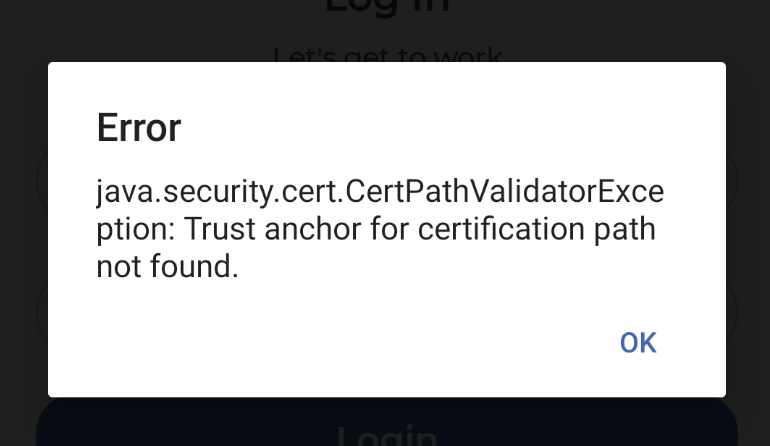
For solve this is issue, you need to ignore the SSL hostname verifier on Android, so you need to create a custom service for this.
- Create the
IHttpClientHandler
interface in form project:
//file name: IHttpClientHandler.cs using System; using System.Net.Http; namespace MyMobileApp.Services { public interface IHttpClientHandler { HttpClient GetHttpClient(); } }
- For iOS, just use the default
HttpClient
is ok, so create the iOS service in iOS project as below:
//file name: HttpClientiOS.cs using System; using System.Net.Http; using System.Runtime.CompilerServices; using MyMobileApp.iOS.Services; using MyMobileApp.Services; using Xamarin.Forms; [assembly: Xamarin.Forms.Dependency(typeof(HttpClientiOS))] namespace MyMobile.iOS.Services { public class HttpClientiOS : IHttpClientHandler { public HttpClient GetHttpClient() { var client = new HttpClient(); return client; } } }
- Create the android service to ignore the
SSL
hostname verifier:
//file name: HttpClientAndroid.cs using System; using System.Net.Http; using Android.Net; using Javax.Net.Ssl; using Org.Apache.Http.Client; using MyMobile.Droid.Services; using MyMobile.Services; using Xamarin.Android.Net; using Xamarin.Forms; [assembly: Dependency(typeof(HttpClientAndroid))] namespace MyMobile.Droid.Services { public class HttpClientAndroid : IHttpClientHandler { public HttpClient GetHttpClient() { var client = new HttpClient(new IgnoreSSLClientHandler()); return client; } } internal class IgnoreSSLClientHandler : AndroidClientHandler { protected override SSLSocketFactory ConfigureCustomSSLSocketFactory(HttpsURLConnection connection) { return SSLCertificateSocketFactory.GetInsecure(1000, null); } protected override IHostnameVerifier GetSSLHostnameVerifier(HttpsURLConnection connection) { return new IgnoreSSLHostnameVerifier(); } } internal class IgnoreSSLHostnameVerifier : Java.Lang.Object, IHostnameVerifier { public bool Verify(string hostname, ISSLSession session) { return true; } } }
4. Use the service in form project:
using (var client = DependencyService.Get<Services.IHttpClientHandler>().GetHttpClient()) { //do the logic for call API var jsonRequest = new { email = this.Email, password = this.Password }; var serializedJsonRequest = JsonConvert.SerializeObject(jsonRequest); HttpContent content = new StringContent(serializedJsonRequest, Encoding.UTF8, "application/json"); var response = await client.PostAsync(new Uri(Globals.APIs.login), content); if (response.IsSuccessStatusCode) { //todo... } }
It’s done!
Views: 22
Total Views: 963 ,